Summary
Asynchronous code does not require the rest of your code to be asynchronous. There are a lot of programs that would be improved with the presence of async, that don’t use it because people are scared of it.
If you have 100 files in your program, and one of those files uses async, you have to either write the entire program in async or resort to bohemian, mind-bending hacks to contain it. If you want to see a good example of a leaky abstraction, consider AppKit.
Non-async code (or “blocking” code) is the real leaky abstraction. I’d like to discuss how you call blocking code from async code, and vice versa.
Futures_lite is not the heaviest dependency on the block (that’s futures_lite), but it’S still a non-negligible amount of code. There’ s also pollster, which has zero (required) dependencies and consists of less than 100 lines of code and consists. of less. than 100 Lines of Code.
The libraries are able to pick up on this runtime and use it. For any block_on calls in your application, the runtime will already be available. Note that you will need to call enter() on any new threads that use tokio primitives.
Async code is more predictable than blocking code, in a weird way. Look at this function signature: fn my_async_function() { /... / } What does this tell you? I know that the Future returned by this function won’t block. I can place it in my executor of choice, or race it against any other Futures. Meanwhile, for blocking code…
Smol provides the blocking threadpool to run code on other threads, while tokio has a spawn_blocking function. But the docs usually fail to mention any of the above behavior, even for functions in the standard library.
I know this is a common complain with tokio’s style of async/await, but it’S just as bad the other way as well. In order to avoid these issues I often have to segment out code that might block into their own sections. This lets me avoid the overhead of unblock for eachfunction as a bonus.
I hope you found this helpful and that it might remove some of the problems. I don’t think it’s a good idea to use the same code over and over again.
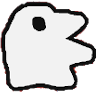