Summary
This series is meant as an introduction to compute shaders. In the next chapter I will provide a small template to run compute shader with. If these topics are still difficult or unknown to you don’t worry. You will likely still be able to follow along with this chapter.
Compute shaders are a small program that we can run on our graphics card thanks to Graphics APIs. A compute shader is a program that can do anything we want it to do. This will likely not be the ‘optimal’ way to use them. Instead, this will be a starting ground to work off from with further research.
With compute shaders we are able to run many complex algorithms and simulations very efficiently. This is called parallel processing. With parallel processing, we can execute our rendering code at rapid speed.
C++ code shows how a single thread has to do a thousand things every frame. Now let’s take the same code and move it into a compute shader. It looks quite similar in many places but this code will likely be significantly faster. Why? Because right now a thousand threads on the GPU will help compute this code at once compared to a single. thread doing a thousand tasks.
Not all code fits parallel computing. Your data needs to be available on the GPU. Uploading or reading back GPU resources can sometimes be an elaborate process.
Optimizing compute shaders can be tricky. Balancing your shaders for maximum thread occupancy can be quite a process to get done right. Determining the right amount of threads and thread groups can also be a thing to balance out.
Dispatch can be seen as a “list of work orders to go through” We tell our GPU that we want to execute our work order X Y Z amount of times. Depending on your GPU, the amount of in-flight work orders/threads can differ. The maximum amount of dispatches per dimension is 65.535^3.
The ‘Group’ in the name means ‘group’ and ‘id’. The ‘D’ stands for ‘determined’
The size of our thread group is just one. This way we can set every pixel in our texture to black. Of course, there are many other ways to do this.
This is most of the theory you need to know about compute shaders to fully begin implementing them. This applies to almost any Graphics API and most engines like Unity or Unreal. The setup per engine/API might be different, but the theory we discussed will mostly be the same.
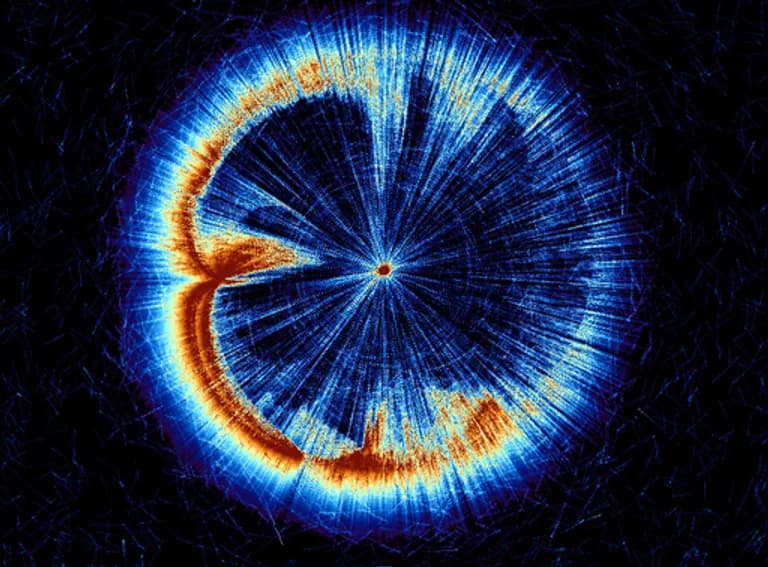