Summary
Go is an open-source programming language created by Google engineers in 2009. Go emphasizes simplicity, performance, and ease of use. It is one of the most popular languages for cloud computing, distributed systems, and large-scale backend services.
Go offers performance on par with lower-level languages like C and C++. Its clean and minimalist syntax allows developers to write, read, and maintain code more efficiently.
Before writing any code, it’s crucial to understand how Go organizes its workspace. You’ll need to set two key environment variables: GOROOT and GOPATH. The GORoOT variable points to the directory where Go is installed, while GOPATH is where your Go projects and dependencies will be stored.
In Go, every program starts with a package declaration. The entry point for any Go application is the main package and the main function. Once your Go code is compiled into a binary, it will be stored here.
Go borrows features from languages like C and Pascal while reducing complexity. It does not require explicit use of semicolons to terminate statements. Go also eliminates some of the more cumbersome features of other languages.
Declaring variables in Go can be done in several ways. The standard method uses the var keyword, followed by the variable name and its type. Go also supports type inference, meaning that if you assign a value to a variable, Go will automatically infer the type.
Go is a programming language with built-in functions for manipulating strings, such as concatenation and slicing. The for loop can be used as a replacement for both for and while loops, making it a highly flexible tool for iterating over data.
Switch statements in Go are also slightly different from other languages. By default, Go’s switch does not fall through to the next case. This makes switch cases cleaner and less error-prone.
Go allows you to use multiple values, which can be used to return both a result and an error. This explicit error handling model is a core feature of Go. Go also supports first-class functions, meaning functions can be assigned to variables, passed as arguments, and returned from other functions.
Every Go program is made up of packages, and the standard library itself is organized into packages. A package is essentially a collection of related Go source files, and it helps organize code in a logical, reusable manner. For example, the fmt package contains functions for formatting text, while the net/http package provides tools for building web servers.
Concurrency is crucial for building scalable applications. Go has a simple yet powerful concurrency model built directly into the language. At the heart of Go’s concurrency are goroutines, lightweight threads managed by the Go runtime.
Go’s approach to error handling is both unique and pragmatic. Unlike many other programming languages that use exceptions for error handling, Go uses a more straightforward model. In Go, errors are treated as explicit return values, and it’re up to the programmer to check these values. This approach promotes transparency and forces developers to think about error handling from the start, leading to more robust and predictable code.
In Go, every error is explicit, making the code more predictable and easier to debug. This approach also avoids the complexities of exception handling found in other languages.
Go’s HTTP package is highly efficient, and when combined with Go’S native support for concurrency via goroutines, it becomes a powerful tool for building scalable web applications.
For developers just getting started, Go offers an accessible learning curve. For more experienced developers, it provides the performance and concurrency features needed to build complex systems.
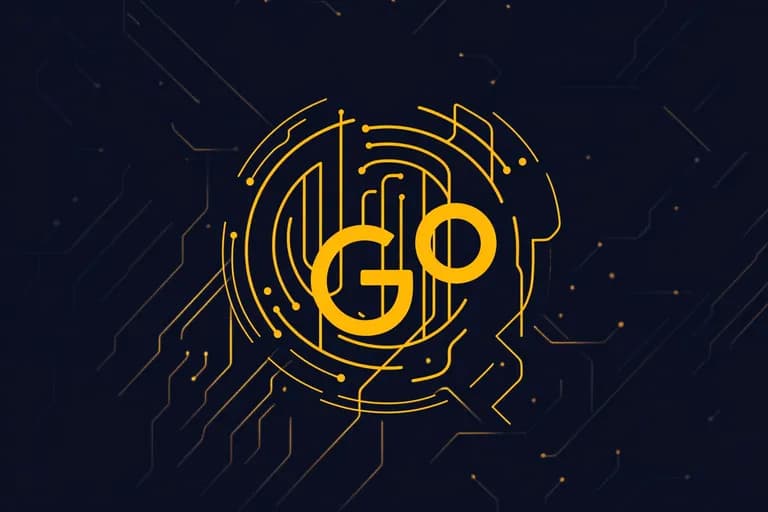