Summary
Programmers working in different industries are divided by a common language. Topics like code clarity, performance, debuggability, architecture and maintainability are a source of friction. Games don’t distribute their source code or interface with the world’s code so certain API restrictions don‘t exist. Games care about performance in many more areas than others.
Compile times are not unique to games, but it is the reality in every large codebase I’ve worked on. It distracts from doing meaningful work and breaks concentration. A full rebuild of “the shaders” can also take a really long time.
The STL’s usage of templates is essentially infinite, a non-trivial language on top of C++. Overusing it is trivial and undoing it not easy. The more people you have making shaders, the more you’ll have to compile.
Games are so dynamic, they tend to spawn and destroy things left and right, be it particles, debris from destruction, short-lived sounds, network packets, etc. In rendering specifically, every frame we prepare and discard thousands of rendering commands, short.lived vertices or per-frame constant buffers.
Casey’s performance video sparked a lot of conversation. It compares a vtable model against a switch for polymorphism and measures why virtual functions can be the wrong tool for a set of problems. Because the language doesn’t provide value types, even vector math and string processing would keep the heap/garbage collector active and hitching all the time.
The last problem caused by the code above was moving it onto the GPU. Since emitters are processed separately we are forced to interleave simulation and rendering. Toggling compute and graphics is very costly and inefficient. The solution would have been to group all related particles into batched compute jobs.
The snippets above follows the chronological order in which these facilities were introduced into C++. Let’s evaluate each option:Standard loop: simple to read, maybe int vs size_t can alter the assembly. Ranged for: perhaps clearest in terms of intent, just uses iterators under the hood.
Don’t worry about code beauty or conciseness (fewer lines of code) Worrying about complex function stacks and lots of little functions is a futile exercise if the tradeoff is a non-trivial cognitive load when reading the code and often worse performance. Try not to reuse variables. If you comment something out in the middle, all the intermediate state is lost.Document your intention (the action is the code itself) and don't be noisy.
Debug build is not the one you hesitantly reach for when you get a bug, it is the default for programmers during development. Not all debug builds are made equal, sometimes you can mix and match. The STL can be very slow in debug builds, and performance can be unpredictable across implementations.
The code in many STL implementations is fairly esoteric and hard to read, and I don’t think there’s good reasons for it. C++ is ultimately a systems programming language that caters to many users, and should be much more dynamic in providing language features.
Graphics programmers need a particular set of tools to analyze and debug: frame analyzers. These are really important as they show what the GPU is up to in a linear, visual way. Using these can be involved depending on what you’re doing. On mobile the landscape is even more fragmented, as each vendor has its own tool.
If your main job is not to be a tools programmer you’ll eventually need to pay attention and expose UIs in ways that make sense. The other side of tools is how fast I can find and invoke the command I’m looking for, e.g. is my UI well organized, does it have clear labels, identifiable buttons and tooltips.
Code versioning tools like Perforce or Git have the ability to start tracking through specific lines of code until their first appearance. I’ve seen practices in some studios that completely disregard this and nuke codebase history. This means losing all the rich history of the codebase.
For more information on how to get your hands on the latest version of this article click here. For more information about how to use the new version of the article, click here: http://www.dailymail.co.uk/news/article-363652/How-to-get-the-latest-version-of-this-article-from-the dailymail and how-to use the-new-version of the article from the_daily weekend.
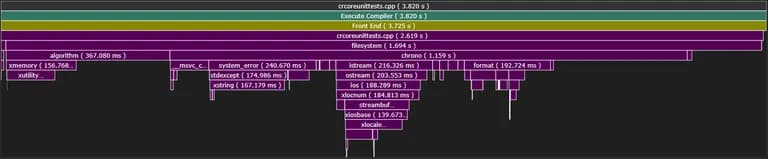