Summary
When you render HTML, the live view of those rendered elements in the browser is called the DOM. There is an entire set of APIs specifically dealing with modifying this tree of elements. These are attached to the document, so you use them like const el = document.querySelector("#el"); They are also available on all other elements, so if you have an element reference you can use these methods.
Visual Studio Code is written in vanilla JavaScript “to be as close to the DOM as possible” This article explains how to keep your DOM unchanged by hiding and showing elements instead of destroying and creating them with JavaScript.
The insertAdjacentHTML method is much faster than innerText because it doesn’t have to destroy the DOM first before inserting. The innerText method is cool because it is aware of the current styles of an element. If you want an element both visually hidden and hidden to assistive technology, display: none; should do it.
When you remove a DOM node, you don’t want references sitting around that prevent the garbage collector from cleaning up associated data. You can associate data to DOM nodes using WeakMap.
Using matches(selector) only matches the current element, so it needs to be the leaf node. You can use the Abort controller to remove sets of events.
Memory profiling can help you identify potential leaks or unnecessary allocations during DOM manipulation. The Performance tab is invaluable for analyzing JavaScript execution time, which is crucial when optimizing DOM manipulation code.
Understanding and applying these low-level techniques can significantly boost your app's performance. Excessive manipulation, even with efficient methods, can still lead to performance issues. The goal isn’t always to forgo frameworks and manually manipulate the DOM for every project. Rather, it’s to understand these principles so you can make informed decisions about when to use frameworks and when to optimize at a lower level.
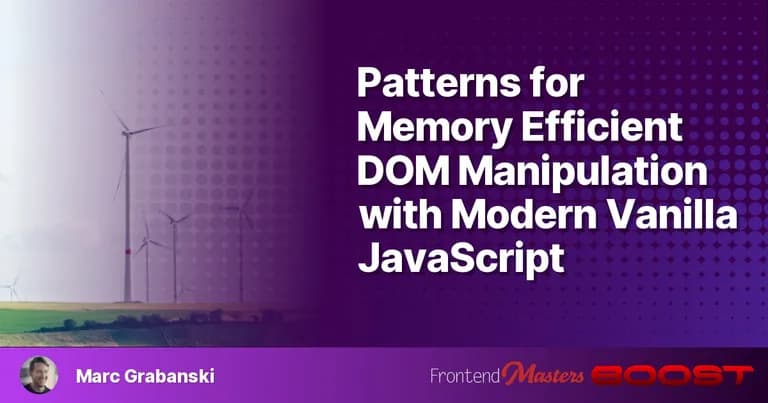